Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Serialization
Python provides built-in JSON libraries to encode and decode JSON.
In Python 2.5, the simplejson module is used, whereas in Python 2.7, the json module is used. Since this interpreter uses Python 2.7, we'll be using json.
In order to use the json module, it must first be imported:
There are two basic formats for JSON data. Either in a string or the object datastructure. The object datastructure, in Python, consists of lists and dictionaries nested inside each other. The object datastructure allows one to use python methods (for lists and dictionaries) to add, list, search and remove elements from the datastructure. The String format is mainly used to pass the data into another program or load into a datastructure.
To load JSON back to a data structure, use the "loads" method. This method takes a string and turns it back into the json object datastructure:
To encode a data structure to JSON, use the "dumps" method. This method takes an object and returns a String:
Python supports a Python proprietary data serialization method called pickle (and a faster alternative called cPickle).
You can use it exactly the same way.
The aim of this exercise is to print out the JSON string with key-value pair "Me" : 800 added to it.
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
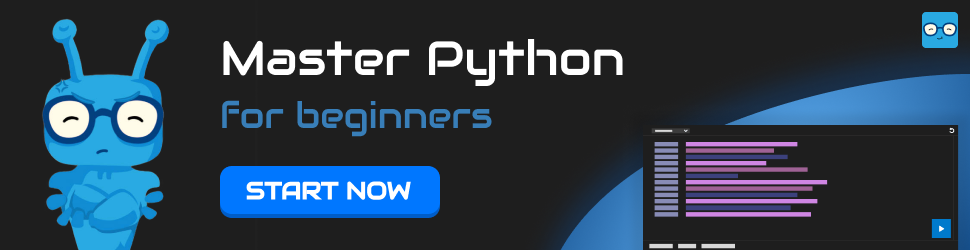